From the Trenches: Automating JWT OAuth with Postman
Postman is a collaboration platform for API development used by many Docusign developers to test Docusign API functions in demo environments. Many of those same developers choose the JSON Web Token (JWT) Grant authentication flow to replace legacy authentication methods, based on the X-Docusign-Authentication header, in their existing Docusign apps because legacy authentication will soon no longer be supported.
This raises an awkward development scenario. At the moment, to make the JWT Grant authorization flow work with Postman, developers often resort to generating the access token using an SDK, or sometimes manually using Curl functions. Then the token value is copied into Postman against the API function to be run, using the header: Authorization: Bearer <ACCESS_TOKEN>
.
Developers need a straightforward way to generate the access token directly in Postman without having to juggle with another tool. The solution I’m describing in this post uses pre-request scripts in Postman to execute JavaScript before the HTTP API request runs. I’ll also embed a JavaScript cryptography library inside a variable value, allowing me to import crypto functions that I will use to handle the private key and create the encoded assertion.
Step 1: Start with the Docusign Postman Collections
I’ll use the already-implemented Postman request “Docusign REST API > Authentication > 02 JWT Access Token” available in the Docusign Postman collections.
This request takes as input the encoded assertion that I must provide:

The request returns the JWT access token through the Docusign REST API “POST https://{{hostenv}}/oauth/token” and assigns it to an environment variable accessToken
:

Step 2: Embed the crypto library and private key in environment variables
I'll use the open-source free JavaScript cryptography library jsrsasign. Then I’ll create an environment variable, jsrsasign
, with its value assigned from the library content:
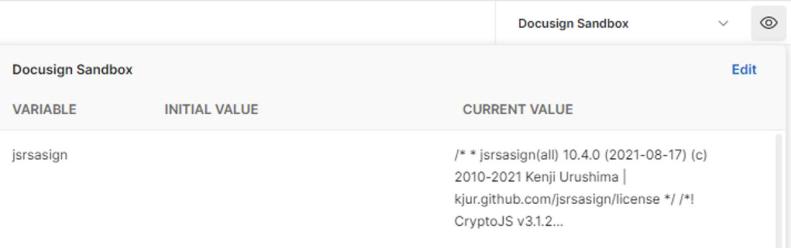
I’ll create another environment variable, jwk
, to store (in Base64 format) the private key for the app, which is generated in your Docusign account on the Apps and Keys page.

Step 3: Build the pre-request script
For this example, some values are directly hard-coded in the assertion body (including iss
, sub
, aud
, and scope
), but you could also use environment variables to implement them, of course.
// Loading the jsrsasign library into Postman Sandbox
var navigator = {}; // Fake a navigator object for the lib
var window = {}; // Fake a window object for the lib
eval(pm.environment.get("jsrsasign")); // Import JavaScript jsrsasign
var timestamp = new Date(); // The current time in milliseconds
var issuedAtTimeSeconds = timestamp/1000;
var expirationTimeSeconds = timestamp/1000 + 3600;
// Create header and payload objects
var header = {
"typ": "JWT",
"alg": "RS256"
};
var payload = {
"iss": "<INTEGRATION_KEY>",
"sub": "<USERID>",
"iat" : Math.ceil(issuedAtTimeSeconds),
"exp" : Math.ceil(expirationTimeSeconds),
"aud": "account-d.docusign.com",
"scope": "signature impersonation"
};
// Prep the objects for a JWT
var sHeader = JSON.stringify(header);
var sPayload = JSON.stringify(payload);
var jwk = pm.environment.get("jwk");
var prvKey = KEYUTIL.getKey(jwk);
var sJWT = KJUR.jws.JWS.sign(header.alg, sHeader, sPayload, prvKey);
pm.environment.set("assertionHere", sJWT);
Now the Postman request is ready to be run. And it will be needed to run it again every time the access token has expired.