Common API Tasks🐈: Deactivate (or close) a user in the Docusign account
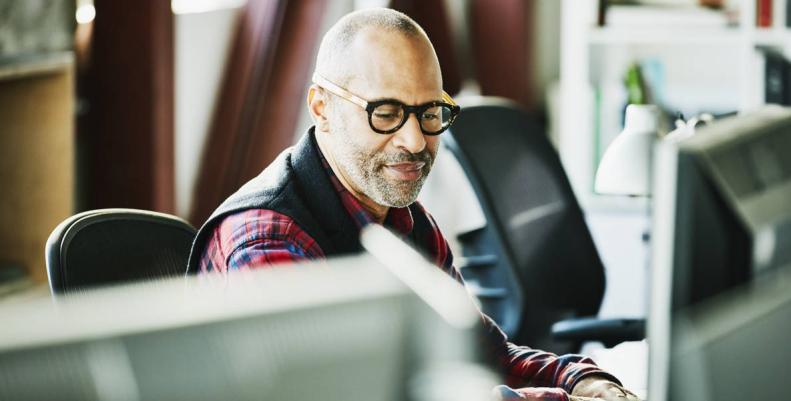
Welcome to a tremendous new edition of the CAT🐈 (Common API Tasks) blog series. The CAT blogs provide all you need to complete small, specific, SDK-supported tasks using one of our APIs. You can find all articles in this series on the Docusign Developer Blog.
Three years ago, I wrote an article in this series showing you how to add users to the Docusign account. In this post I’m going to show you how you can write code that closes or deactivates (in essence, removes) a user from a Docusign account. As a refresher, I will remind you that an account in Docusign can have many users. Each of these users have membership in that account and they can also have membership in other accounts. Furthermore, accounts can be grouped into organizations. Here is a quick graph to show the relationship between users, members, accounts, and organizations:
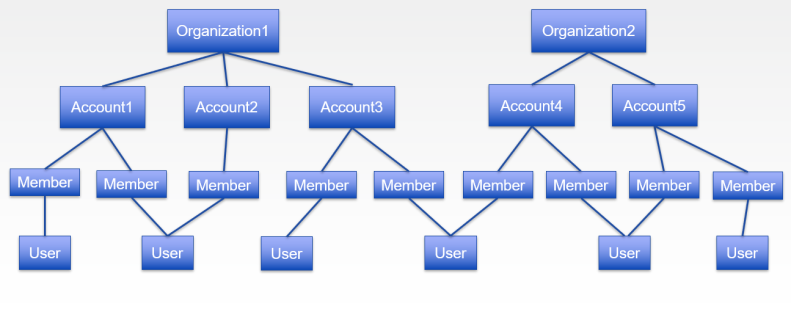
What I’m demonstrating in this blog post is closing a membership in a specific account; or, in other words, deactivating the user from that account. A couple of notes:
- This operation is reversible. You can reactivate a user, but that user will have to click a link in an email message to indicate that they agree to be active again.
- You must be an administrator of the account to have the right to perform this action. Only users with administrative privileges can remove other users. This means the access token provided for the API calls below must be from such a user.
And now, code. This is a simple code snippet that first looks up the user you want to remove, and then, in the second API call, removes it. Note that the code searches for the user based on their email address, but you can also search based on name or any other property accessible through the Users::list API call.
C#
DocuSignClient docuSignClient = new DocuSignClient(basePath);
// You will need to obtain an access token using your chosen authentication method
docuSignClient.Configuration.DefaultHeader.Add("Authorization", "Bearer " + accessToken);
UsersApi usersApi = new UsersApi(docuSignClient);
// Find the user to deactivate/close
UsersApi.ListOptions listOptions = new UsersApi.ListOptions();
listOptions.emailSubstring = "email@domain.com";
UserInformationList userInformationList = usersApi.List(accountId, listOptions);
// Make the request to “delete” that particular user
UserInfoList userInfoList = new UserInfoList();
userInfoList.Users = new List<UserInfo> { new UserInfo { UserId = userInformationList.Users[0].UserId }};
usersApi.Delete(accountId, userInfoList);
Java
Configuration config = new Configuration(new ApiClient(basePath));
// You will need to obtain an access token using your chosen authentication method
config.addDefaultHeader("Authorization", "Bearer " + accessToken);
UsersApi usersApi = new UsersApi(apiClient);
// Find the user to deactivate/close
UsersApi.CallListOptions listOptions = usersApi.new CallListOptions();
listOptions.setEmailSubstring("email@domain.com");
UserInformationList userInformationList = usersApi.callList(accountId, listOptions);
// Make the request to “delete” that particular user
UserInfoList userInfoList = new UserInfoList();
java.util.ArrayList<UserInfo> users = new java.util.ArrayList<UserInfo>();
UserInfo user = new UserInfo();
user.setUserId(userInformationList.getUsers().get(0).getUserId());
users.add (user);
userInfoList.setUsers(users);
usersApi.delete(accountId, userInfoList);
Node.js
let dsApiClient = new docusign.ApiClient();
dsApiClient.setBasePath(basePath);
// You will need to obtain an access token using your chosen authentication method
dsApiClient.addDefaultHeader('Authorization', 'Bearer ' + accessToken);
let usersApi = new docusign.UsersApi(dsApiClient);
// Find the user to deactivate/close
let userInformationList = usersApi.list(accountId, {'email_substring' : 'email@domain.com'} );
// Make the request to “delete” that particular user
let userInfoList = new docusign.UserInfoList();
let userInfo = new docusign.UserInfo();
userInfo.userId = userInformationList.users[0].userId;
userInfoList.users = [ userInfo ];
usersApi.delete(accountId, userInfoList);
PHP
$api_client = new \Docusign\eSign\client\ApiClient($base_path);
$config = new \Docusign\eSign\Model\Configuration($api_client);
# You will need to obtain an access token using your chosen authentication method
$config->addDefaultHeader('Authorization', 'Bearer ' + $access_token);
$users_api = new \Docusign\eSign\Api\UsersApi($api_client);
# Find the user to deactivate/close
$list_options = new \Docusign\eSign\Api\UsersApi\ListOptions();
$list_options->setEmailSubstring('email@domain.com');
$user_information_list = $users_api->callList($account_id, $list_options);
# Make the request to “delete” that particular user
$user_info_list = new \Docusign\eSign\Model\UserInfoList();
$user_info = new \Docusign\eSign\Model\UserInfo();
$user_info->setUserId($user_information_list->getUsers()[0]->getUserId());
$users = [$user_info];
$user_info_list->setUsers($users);
$users_api->delete($account_id, $user_info_list);
Python
api_client = ApiClient()
# You will need to obtain an access token using your chosen authentication method
api_client.set_default_header('Authorization', 'Bearer ' + access_token)
users_api = UsersApi(api_client)
# Find the user to deactivate/close
user_information_list = users_api.list(account_id, email_substring = 'email@domain.com')
# Make the request to “delete” that particular user
user_info_list = UserInfoList()
user_info = UserInfo()
user_info.user_id = user_information_list.users[0].user_id
user_info_list.users = [ user_info ]
users_api.delete(account_id, user_info_list)
Ruby
config = DocuSign_eSign::Configuration.new
config.host = base_path
api_client = DocuSign_eSign::ApiClient.new config
# You will need to obtain an access token using your chosen authentication method
api_client.DefaultHeader['Authorization'] = 'Bearer ' + access_token
users_api = DocuSign_eSign::UsersApi.new api_client
# Find the user to deactivate/close
list_options = DocuSign_eSign::ListOptions.new
list_options.email_substring = 'email@domain.com'
user_information_list = users_api.list(account_id, list_options)
# Make the request to “delete” that particular user
user_info_list = DocuSign_eSign::UserInfoList.new
user_info = DocuSign_eSign::UserInfo.new
user_info.user_id = user_information_list.users[0].user_id
user_info_list.users = [ user_info ]
users_api.delete(account_id, user_info_list)
That’s all, folks! I hope you found it useful. If you have any questions, comments, or suggestions for topics for future Common API Tasks posts, feel free to email me. Until next time...